|
|
Title | Find solutions to the equilateral triangle puzzle in Visual Basic .NET |
Description | This example shows how to find solutions to the equilateral triangle puzzle in Visual Basic .NET. |
Keywords | algorithms, games, graphics, mathematics, example, example program, Windows Forms programming, Visual Basic .NET, VB.NET |
Categories | Algorithms, Algorithms, Puzzles and Games |
|
|
This program uses the following code to find the solutions to the puzzle Puzzle: find the equilateral triangles in Visual Basic .NET.
|
|
' Find the solutions.
Private Sub btnFindSolutions_Click(ByVal sender As _
System.Object, ByVal e As System.EventArgs) Handles _
btnFindSolutions.Click
Cursor = Cursors.WaitCursor
Solutions = New List(Of Integer())()
For i As Integer = 0 To Points.Length - 1
For j As Integer = i + 1 To Points.Length - 1
Dim dx_ij As Double = Points(j).X - Points(i).X
Dim dy_ij As Double = Points(j).Y - Points(i).Y
Dim dist_ij As Double = Math.Sqrt(dx_ij * dx_ij _
+ dy_ij * dy_ij)
For k As Integer = j + 1 To Points.Length - 1
Dim dx_jk As Double = Points(k).X - _
Points(j).X
Dim dy_jk As Double = Points(k).Y - _
Points(j).Y
Dim dist_jk As Double = Math.Sqrt(dx_jk * _
dx_jk + dy_jk * dy_jk)
Const tiny As Double = 0.0001
If (Math.Abs(dist_ij - dist_jk) < tiny) Then
Dim dx_ki As Double = Points(i).X - _
Points(k).X
Dim dy_ki As Double = Points(i).Y - _
Points(k).Y
Dim dist_ki As Double = Math.Sqrt(dx_ki _
* dx_ki + dy_ki * dy_ki)
If (Math.Abs(dist_jk - dist_ki) < tiny) _
Then
' This is a solution.
Solutions.Add(New Integer() {i, j, _
k})
End If
End If
Next k
Next j
Next i
lblNumSolutions.Text = Solutions.Count.ToString() & "" & _
"solutions"
btnFindSolutions.Enabled = False
btnShowSolutions.Enabled = True
btnShowAllSolutions.Enabled = True
Refresh()
Cursor = Cursors.Default
End Sub
|
|
The code loops through all of the points three times. Each loop starts at the point after the point used in the enclosing loop so the innermost loop only considers each triple of points once and the points in each triple are unique. In other words, the code doesn't look at triples that contain a point more than once.
The code calculates the distances between the pairs of points in each triple and, if the distances are the same, adds a new triangle holding the points to the solution list.
Note that the distances are floating point values and rounding errors often make floating point values not exactly equal when they should be the same. This is a common problem when working with floating point numbers that should be the same. To avoid problems with equality testing, the code subtracts two distances, takes the absolute value, and checks whether the result is close to 0.
For example, when testing some of the points, the program finds these values that should be equal:
76.210235595703125
76.210235548698734
Subtracting them gives 0.000000047004391490190756, which is smaller than the value tiny defined by this code as 0.0001, so the program treats the two values as equal. (In fact, this example is measuring distances in pixels so you could define tiny to be 1 and you would still find the correct solutions.)
Related links:
|
|
|
|
|
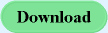 |
|
|
|