|
|
Title | Drag and drop images while displaying a preview in Visual Basic .NET |
Description | This example shows how to drag and drop images while displaying a preview in Visual Basic .NET. |
Keywords | drag, drop, graphics, drag and drop preview, drag and drop, drag and drop image, drag and drop image preview, VB.NET, Visual Basic .NET |
Categories | Controls |
|
|
The example Drag and drop images in Visual Basic .NET explains how to drag and drop images. See that example for the basic ideas. (Pay special attention to the first paragraph, which discusses the AllowDrop property.)
This example adds a preview to the drop. When you drag an image over the drop target, it displays a grayed out version of the image.
When you right-click on a drag source PictureBox, the following code starts the drag.
|
|
' Start the drag.
Private Sub picDragSource_MouseDown(ByVal sender As _
System.Object, ByVal e As _
System.Windows.Forms.MouseEventArgs) Handles _
picDragSource.MouseDown, picDragSource2.MouseDown
' Start the drag if it's the right mouse button.
If (e.Button = MouseButtons.Right) Then
Dim source As PictureBox = DirectCast(sender, _
PictureBox)
picDragSource.DoDragDrop(source.Image, _
DragDropEffects.Copy)
End If
End Sub
|
|
This code calls DoDragDrop to start the drag, passing it the image to drag.
When the drag moves over the drop target, the following code executes.
|
|
' The current image during a drag.
Private OldImage As Image = Nothing
' Allow a copy of an image.
Private Sub picDropTarget_DragEnter(ByVal sender As _
System.Object, ByVal e As _
System.Windows.Forms.DragEventArgs) Handles _
picDropTarget.DragEnter
' See if this is a copy and the data includes an image.
If (e.Data.GetDataPresent(DataFormats.Bitmap) AndAlso _
(e.AllowedEffect And DragDropEffects.Copy) <> 0) _
Then
' Allow this.
e.Effect = DragDropEffects.Copy
' Save the current image.
OldImage = picDropTarget.Image
' Display the preview image.
Dim bm As Bitmap = _
DirectCast(e.Data.GetData(DataFormats.Bitmap, _
True), Bitmap)
Dim copy_bm As Bitmap = DirectCast(bm.Clone(), _
Bitmap)
Using gr As Graphics = Graphics.FromImage(copy_bm)
' Cover with translucent white.
Using br As New SolidBrush(Color.FromArgb(128, _
255, 255, 255))
gr.FillRectangle(br, 0, 0, bm.Width, _
bm.Height)
End Using
End Using
picDropTarget.Image = copy_bm
Else
' Don't allow any other drop.
e.Effect = DragDropEffects.None
End If
End Sub
|
|
If Bitmap data is present and this is a copy operation, the code allows the copy. It saves the drop target's current image. It then makes a copy of the dragged image and draws a translucent white rectangle over it to make it lighter. It then displays the preview image in the drop target.
If the drag leaves the drop target, the following code executes.
|
|
' Remove the drag enter image.
Private Sub picDropTarget_DragLeave(ByVal sender As _
System.Object, ByVal e As System.EventArgs) Handles _
picDropTarget.DragLeave
' Restore the saved image.
picDropTarget.Image = OldImage
End Sub
|
|
This code restores the drop target's original image.
Finally if the user drops on the target, the following code executes.
|
|
' Accept the drop.
Private Sub picDropTarget_DragDrop(ByVal sender As _
System.Object, ByVal e As _
System.Windows.Forms.DragEventArgs) Handles _
picDropTarget.DragDrop
Dim bm As Bitmap = _
DirectCast(e.Data.GetData(DataFormats.Bitmap, True), _
Bitmap)
picDropTarget.ClientSize = bm.Size
picDropTarget.Image = bm
End Sub
|
|
This code displays the dropped image and makes the PictureBox fit the image.
Initially I set the drop target's AutoSize property to AutoSize so it would fit the image. This caused some weird flickering problems when you dragged images of different sizes over the control. Give it a try and see. This version is much better.
With a little extra work, you could drag the image to a specific position on the drop target. In that case, you could display a preview of the image in its new position.
|
|
|
|
|
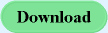 |
|
|
|