Title | Drag and drop images in Visual Basic .NET |
Description | This example shows how to drag and drop images in Visual Basic .NET. |
Keywords | drag, drop, graphics, drag and drop, drag and drop image, VB.NET, Visual Basic .NET |
Categories | Controls |
|
|
One of the first things you must do before implementing drag and drop is set the AllowDrop property to true for the control that should accept a drop. For some reason, Visual Studio does not display this property in the properties window for PictureBoxes. IntelliSense doesn't even admit the property exists!
When you right-click on a drag source PictureBox, the following code starts the drag.
|
|
' Start the drag.
Private Sub picDragSource_MouseDown(ByVal sender As _
System.Object, ByVal e As _
System.Windows.Forms.MouseEventArgs) Handles _
picDragSource.MouseDown, picDragSource2.MouseDown
' Start the drag if it's the right mouse button.
If (e.Button = MouseButtons.Right) Then
Dim source As PictureBox = DirectCast(sender, _
PictureBox)
picDragSource.DoDragDrop(source.Image, _
DragDropEffects.Copy)
End If
End Sub
|
|
When the drag moves over the drop target, the following code executes.
|
|
' Allow a copy of an image.
Private Sub picDropTarget_DragEnter(ByVal sender As _
System.Object, ByVal e As _
System.Windows.Forms.DragEventArgs) Handles _
picDropTarget.DragEnter
' See if this is a copy and the data includes an image.
If (e.Data.GetDataPresent(DataFormats.Bitmap) AndAlso _
(e.AllowedEffect And DragDropEffects.Copy) <> 0) _
Then
' Allow this.
e.Effect = DragDropEffects.Copy
Else
' Don't allow any other drop.
e.Effect = DragDropEffects.None
End If
End Sub
|
|
If Bitmap data is present and this is a copy operation, the code allows the copy.
Finally if the user drops on the target, the following code executes.
|
|
' Accept the drop.
Private Sub picDropTarget_DragDrop(ByVal sender As _
System.Object, ByVal e As _
System.Windows.Forms.DragEventArgs) Handles _
picDropTarget.DragDrop
Dim bm As Bitmap = DirectCast( _
e.Data.GetData(DataFormats.Bitmap, True), Bitmap)
picDropTarget.Image = bm
End Sub
|
|
This code sets the drop target's Image property equal to the dropped bitmap. The drop target PictureBox has ScaleMode set to AutoSize so it resizes itself to fit the dropped image.
|
|
|
|
|
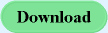 |
|