Title | Draw a fractal that is generated by applying rules to prime numbers in Visual Basic 6 |
Description | This example shows how to draw a fractal that is generated by applying rules to prime numbers in Visual Basic 6. |
Keywords | graphics, fractals, primes, prime numbers, Visual Basic 6, VB 6 |
Categories | Graphics, Graphics |
|
|
(For information and discussion of this idea, see The prime-explosion Bergot graph at The Prime Puzzles & Problem Connection.)
The program draws the image by following these rules:
- Start at the point (0, 0) corresponding to the prime 2.
- Go to the next prime.
- If the prime is 5, ignore it. Otherwise the prime has one of these forms:
- 5n + 1: Move up 1 pixel
- 5n + 2: Move right 1 pixel
- 5n + 3: Move down 1 pixel
- 5n + 4: Move left 1 pixel
- Repeat at step 2 until bored.
Periodically the program makes a bitmap where the colors of the pixels indicate the number of times each point was visited. The image shown here was generated by plotting 3 million points.
The form's Load event handler creates a Hits array to record the number of times a pixel is visited.
|
|
Private Const Wid As Integer = 800
Private Const Hgt As Integer = 800
Private Const XOff As Integer = 150
Private Const YOff As Integer = 200
Private Hits(Wid, Hgt) As Integer
Private CurX As Integer
Private CurY As Integer
Private CurrentPrime As Long
Private Running As Boolean
Private NumPoints As Long
' Initialize data structures.
Private Sub Form_Load()
CurX = 0
CurY = 0
CurrentPrime = 1
Running = False
NumPoints = 0
picFractal.ScaleMode = vbPixels
End Sub
|
|
When you select the File menu's Start item, the following code executes.
|
|
' Start or stop.
Private Sub mnuFileStart_Click()
If (mnuFileStart.Caption = "&Start") Then
' Start.
Running = True
mnuFileStart.Caption = "&Stop"
DrawFractal
' Clean up after we finish running.
mnuFileStart.Enabled = True
mnuFileStart.Caption = "&Start"
Else
' Stop.
Running = False
mnuFileStart.Enabled = False
End If
End Sub
|
|
If the menu item currently says Start, the program starts calculating. It sets Running to true so it knows it's running, changes the menu item's text to Stop, and calls the DrawFractal method. After that method returns, the code enables the menu item and resets its text to Start.
If the menu item currently doesn't say Start, then the program is currently drawing the fractal. In that case the code sets Running to false and disables the menu item.
The following code shows the DrawFractal method.
|
|
' Add points to the fractal.
Private Sub DrawFractal()
Const points_per_loop As Integer = 10000
Dim i As Integer
Dim ix As Integer
Dim iy As Integer
Do While (Running)
' Generate a bunch of points.
For i = 1 To points_per_loop
' Find the next prime.
CurrentPrime = FindNextPrime(CurrentPrime)
' See which kind of prime it is.
Select Case (CurrentPrime Mod 5)
Case 1
CurY = CurY - 1
Case 2
CurX = CurX + 1
Case 3
CurY = CurY + 1
Case 4
CurX = CurX - 1
End Select
' Record the hit.
ix = CurX + XOff
iy = CurY + YOff
If (ix >= 0 And iy >= 0 And ix < Wid And iy < _
Hgt) Then
Hits(ix, iy) = Hits(ix, iy) + 1
End If
Next i
' Build the image.
BuildImage
' Display the point count.
NumPoints = NumPoints + points_per_loop
lblNumPoints.Caption = NumPoints
lblPrime.Caption = CurrentPrime
' Process button clicks if there were any.
DoEvents
Loop
End Sub
|
|
This code enters a loop that executes as long as Running is true. Inside that loop, the code executes another loop to plot a bunch of points.
The inner loop calls FindNextPrime to get the next prime number. It then uses a switch statement to decide which rule to use and updates the current point accordingly. It then updates the new point's Hits count.
After the inner loop finishes, the program calls BuildImage to display the fractal so far. It then updates a couple labels and calls Application.DoEvents to allow the program to process Windows events. That allows the Start/Stop menu item's event handler to execute if the user selected the item to stop running. That event handler sets Running to false so the outer loop stops.
The FindNextPrime method is straightforward.
|
|
' Find the next prime after this one.
Private Function FindNextPrime(ByVal value As Long) As Long
Dim i As Long
' Cheat a little for speed.
'if (value = 1) return 2
'if (value = 2) return 3
i = value + 2
Do
If (IsPrime(i)) Then
FindNextPrime = i
Exit Do
End If
i = i + 2
Loop
End Function
|
|
The BuildImage method is also straightforward. It clears the PictureBox and then uses the Hits array and PSet to set its pixel values. See the code for the details.
The only non-trivial part of this method is the fact that it uses the MapRainbowColor method to assign colors to the pixels based on their hit values. For a description of this method, see Map numeric values to colors in a rainbow in Visual Basic 6.
|
|
|
|
|
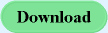 |
|