|
|
Title | Show samples of EXIF image orientations in Visual Basic .NET |
Description | This example shows how to show samples of EXIF image orientations in Visual Basic .NET. |
Keywords | graphics, files, image processing, EXIF, orientation, rotate image, example, example program, Windows Forms programming, Visual Basic .NET, VB.NET |
Categories | Graphics, Graphics, Algorithms |
|
|
This example includes an ExifStuff module that holds routines for working with EXIF data. The following code shows how the module defines the ExifOrientations enumeration.
|
|
' Orientations.
Public Enum ExifOrientations As Byte
Unknown = 0
TopLeft = 1
TopRight = 2
BottomRight = 3
BottomLeft = 4
LeftTop = 5
RightTop = 6
RightBottom = 7
LeftBottom = 8
End Enum
|
|
The following code shows the OrientationImage method, which returns a sample image for each EXIF orientation.
|
|
' Make an image to demonstrate orientations.
Public Function OrientationImage(ByVal orientation As _
ExifOrientations) As Image
Const size As Integer = 64
Dim bm As New Bitmap(size, size)
Using gr As Graphics = Graphics.FromImage(bm)
gr.Clear(Color.White)
gr.TextRenderingHint = _
System.Drawing.Text.TextRenderingHint.AntiAliasGridFit
' Orient the result.
Select Case (orientation)
Case ExifOrientations.TopLeft
Case ExifOrientations.TopRight
gr.ScaleTransform(-1, 1)
Case ExifOrientations.BottomRight
gr.RotateTransform(180)
Case ExifOrientations.BottomLeft
gr.ScaleTransform(1, -1)
Case ExifOrientations.LeftTop
gr.RotateTransform(90)
gr.ScaleTransform(-1, 1, MatrixOrder.Append)
Case ExifOrientations.RightTop
gr.RotateTransform(-90)
Case ExifOrientations.RightBottom
gr.RotateTransform(90)
gr.ScaleTransform(1, -1, MatrixOrder.Append)
Case ExifOrientations.LeftBottom
gr.RotateTransform(90)
End Select
' Translate the result to the center of the bitmap.
gr.TranslateTransform(size / 2, size / 2, _
MatrixOrder.Append)
Using string_format As New StringFormat()
string_format.LineAlignment = _
StringAlignment.Center
string_format.Alignment = StringAlignment.Center
Using the_font As New Font("Times New Roman", _
40, GraphicsUnit.Point)
If (orientation = ExifOrientations.Unknown) _
Then
gr.DrawString("?", the_font, _
Brushes.Black, 0, 0, string_format)
Else
gr.DrawString("F", the_font, _
Brushes.Black, 0, 0, string_format)
End If
End Using
End Using
End Using
Return bm
End Function
|
|
This code simply uses scale and translation transformations to orient its drawing output. It then draws the letter F to produce an image that shows the letter with the correct EXIF orientation.
The main program contains 8 PictureBoxes. When it starts, the program uses the following code to make each PictureBox display one of the EXIF orientation images.
|
|
Private Sub Form1_Load(ByVal sender As System.Object, ByVal _
e As System.EventArgs) Handles MyBase.Load
pic1.Image = OrientationImage(ExifOrientations.TopLeft)
pic2.Image = OrientationImage(ExifOrientations.TopRight)
pic3.Image = _
OrientationImage(ExifOrientations.BottomRight)
pic4.Image = _
OrientationImage(ExifOrientations.BottomLeft)
pic5.Image = OrientationImage(ExifOrientations.LeftTop)
pic6.Image = OrientationImage(ExifOrientations.RightTop)
pic7.Image = _
OrientationImage(ExifOrientations.RightBottom)
pic8.Image = _
OrientationImage(ExifOrientations.LeftBottom)
End Sub
|
|
|
|
|
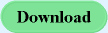 |
|
|
|