|
|
Title | Remove the X Close button from a form's system menu in Visual Basic .NET |
Description | This example shows how to remove the X Close button from a form's system menu in Visual Basic .NET. |
Keywords | system, forms, remove close button, remove system button, remove X button, example example, program, Windows Forms programming, Visual Basic .NET, VB.NET |
Categories | Software Engineering, Controls |
|
|
Visual Basic doesn't have a built-in way to manipulate the system menu that appears when you click the upper left corner of a form, but it's not too hard to use API functions to remove some or all of those buttons.
This example uses the following Imports statement:
|
|
Imports System.Runtime.InteropServices
|
|
This statement allows the program to use the DllImport statement to import the User32.dll library methods in the following code.
|
|
' Declare User32 constants and methods.
Private Const MF_BYPOSITION As Integer = &H400
<DllImport("user32.dll", _
CallingConvention:=CallingConvention.Cdecl)> _
Private Shared Function GetSystemMenu(ByVal hWnd As IntPtr, _
ByVal bRevert As Boolean) As IntPtr
End Function
<DllImport("user32.dll")> _
Public Shared Function GetMenuItemCount(ByVal hMenu As _
IntPtr) As Int32
End Function
<DllImport("user32.dll")> _
Public Shared Function RemoveMenu(ByVal hMenu As IntPtr, _
ByVal nPosition As Int32, ByVal wFlags As Int32) As _
Int32
End Function
' Remove the X button.
Private Sub Form1_Load(ByVal sender As System.Object, ByVal _
e As System.EventArgs) Handles MyBase.Load
Dim hMenu As IntPtr = GetSystemMenu(Me.Handle, False)
Dim num_menu_items As Integer = GetMenuItemCount(hMenu)
'For i As Integer = num_menu_items - 1 To 0 Step -1
' RemoveMenu(hMenu, i, MF_BYPOSITION)
'Next i
RemoveMenu(hMenu, num_menu_items - 1, MF_BYPOSITION)
RemoveMenu(hMenu, num_menu_items - 2, MF_BYPOSITION)
End Sub
|
|
This code first defines the GetSystemMenu, GetMenuItemCount, and RemoveMenu API functions. The form's Load event handler uses GetSystemMenu to get the handle for the form's system menu. It then uses GetMenuItemCount to see how many items the menu contains. It then calls RemoveMenu twice to remove the Close menu item and the separator that comes before it in the menu.
You can see in the picture that the X menu item and the separator before it has been completely removed. If you look very closely, you can also see that the X close button in the form's upper right corner is disabled.
Notice that the code removes the menu's last item first and then removes the second-to-last item second. If it tried to remove the second-to-last item first, then the menu would have one fewer item when the code tried to remove the last item so it wouldn't work. The code should only remove items in positions that exist at the time.
If you remove all of the system's menu items, the user will not be able to move, resize, or close the form, and the form's system menu will not appear. However the system menu's symbol still appears in the form's upper left corner, the cursor still changes to the resize cursor when you hold it over the form's borders, and the minimize and maximize buttons still work. All in all, this is pretty confusing to the user. If you want to remove that menu completely, set the form's ControlBox property to False instead.
Also note that none of these techniques prevent the user from closing the form by pressing Alt+F4.
|
|
|
|
|
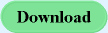 |
|
|
|