|
|
Title | Make a second version of the kaleidoscope program in Visual Basic .NET |
Description | This example shows how to use a list of transformation matrices to make a kaleidoscope program in Visual Basic .NET. |
Keywords | kaleidoscope, drawing, art, graphics, transformations, Visual Basic .NET, VB.NET |
Categories | Graphics |
|
|
This is a revised version of the example Make a kaleidoscope program in Visual Basic .NET. This version works much as the previous version except it uses an array of transformations to draw.
When you click and draw on this program's form, the code draws other curves related to yours. For example, it might draw a mirror image of what you draw or it might repeat your drawing rotated by multiples of 30 degrees.
MouseDown, MouseMove, and MouseUp event handlers do most of the work. The program stores drawing information in a List(Of List(Of Point)). Each item in the list gives a list of points to draw to make a curve. The MouseDown, MouseMove, and MouseUp event handlers create the lists of Points.
The MouseMove event handler refreshes the program's PictureBox, which uses the following event handler to draw your curves and the modified versions.
|
|
' Draw the polylines.
Private Sub picCanvas_Paint(ByVal sender As System.Object, _
ByVal e As System.Windows.Forms.PaintEventArgs) Handles _
picCanvas.Paint
e.Graphics.SmoothingMode = SmoothingMode.AntiAlias
' Make a list of transformations, starting with the
' identity.
Dim matrices As New List(Of Matrix)()
matrices.Add(New Matrix())
' Make transformation matrices for the selected style.
Dim wid As Integer = picCanvas.ClientSize.Width
Dim hgt As Integer = picCanvas.ClientSize.Height
Dim src_rect As New Rectangle(0, 0, wid, hgt)
If (mnuStyleReflectX.Checked OrElse _
mnuStyleReflectXY.Checked) Then
' Reflect across X axis.
Dim pts As Point() = {New Point(wid, 0), New _
Point(0, 0), New Point(wid, hgt)}
Dim mat As New Matrix(src_rect, pts)
matrices.Add(mat)
End If
If (mnuStyleReflectY.Checked OrElse _
mnuStyleReflectXY.Checked) Then
' Reflect across Y axis.
Dim pts As Point() = {New Point(0, hgt), New _
Point(wid, hgt), New Point(0, 0)}
Dim mat As New Matrix(src_rect, pts)
matrices.Add(mat)
End If
If (mnuStyleReflectXY.Checked) Then
' Reflect across X and Y axes.
Dim pts As Point() = {New Point(wid, hgt), New _
Point(0, hgt), New Point(wid, 0)}
Dim mat As New Matrix(src_rect, pts)
matrices.Add(mat)
End If
If (mnuStyleRotate2.Checked) Then
' Rotate 180 degrees.
Dim mat As New Matrix()
mat.RotateAt(180, New PointF(CSng(wid / 2), CSng(hgt _
/ 2)))
matrices.Add(mat)
End If
If (mnuStyleRotate4.Checked) Then
' Rotate 90 degrees three times.
For i As Integer = 1 To 3
Dim mat As New Matrix()
mat.RotateAt(i * 90, New PointF(CSng(wid / 2), _
CSng(hgt / 2)))
matrices.Add(mat)
Next i
End If
If (mnuStyleRotate8.Checked) Then
' Rotate 45 degrees seven times.
For i As Integer = 1 To 7
Dim mat As New Matrix()
mat.RotateAt(i * 45, New PointF(CSng(wid / 2), _
CSng(hgt / 2)))
matrices.Add(mat)
Next i
End If
' Loop through all of the transformations.
For Each mat As Matrix In matrices
e.Graphics.Transform = mat
For Each pline As List(Of Point) In Polylines
e.Graphics.DrawLines(Pens.Blue, pline.ToArray())
Next pline
Next mat
End Sub
|
|
The code makes a List(Of Matrix) to store transformation matrices. It initializes the list by adding the identity transformation, a transformation that leaves the drawing unchanged. Then depending on the kaleidoscope style you selected from the program's menu, the code adds other transformation matrices to the list.
After is has made a list of transformations, the program loops through them. For each transformation, the program loops through the List(Of List(Of Point)) connecting the points to draw the curves you made. The result is several transformed copies of your curves, making a kaleidoscopic result.
|
|
|
|
|
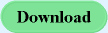 |
|
|
|