|
|
Title | Make an HSet class that inherits from HashSet and that support operators such as union and intersection in Visual Basic .NET |
Description | This example shows how to make an HSet class that inherits from HashSet and that support operators such as union and intersection in Visual Basic .NET. |
Keywords | algorithms, sets, set operations, overloaded operators, HashSet, union, intersection, XOR, Visual Basic .NET, VB.NET |
Categories | Algorithms, Software Engineering |
|
|
The example Use the HashSet class to represent sets and perform set operations in Visual Basic .NET explains how to use the HashSet class to represent and manipulate sets. Unfortunately to manipulate the sets you need to use methods such as IntersectWith, UnionWith, and SymmetricExceptWith instead of more intuitive operators such as And, Or, and Xor.
This example shows how to override those operators to make set operations easier.
To override these operators, you need to add code to the class. You might like to add code directly to the HashSet class by using the partial keyword but that only works if every piece of the class uses the keyword and Microsoft's HashSet class doesn't use that keyword. In other words, you cannot add code to an existing class.
To work around this problem, this example derives a HSet class that inherits from HashSet. This also lets me use the slightly more intuitive class name HSet. (I would have preferred the name Set but that is a reserved word in Visual Basic.)
The following code shows the complete HSet class.
|
|
Public Class HSet(Of T)
Inherits HashSet(Of T)
' Constructors.
Public Sub New()
MyBase.New()
End Sub
Public Sub New(ByVal comparer As IEqualityComparer(Of _
T))
MyBase.New(comparer)
End Sub
Public Sub New(ByVal collection As IEnumerable(Of T))
MyBase.New(collection)
End Sub
Public Sub New(ByVal collection As IEnumerable(Of T), _
ByVal comparer As IEqualityComparer(Of T))
MyBase.New(collection, comparer)
End Sub
Public Sub New(ByVal info As _
System.Runtime.Serialization.SerializationInfo, _
ByVal context As _
System.Runtime.Serialization.StreamingContext)
MyBase.New(info, context)
End Sub
' Union.
Public Shared Operator Or(ByVal A As HSet(Of T), ByVal B _
As HSet(Of T)) As HSet(Of T)
Dim result As New HSet(Of T)(A)
result.UnionWith(B)
Return result
End Operator
' Intersection.
Public Shared Operator And(ByVal A As HSet(Of T), ByVal _
B As HSet(Of T)) As HSet(Of T)
Dim result As New HSet(Of T)(A)
result.IntersectWith(B)
Return result
End Operator
' Xor.
Public Shared Operator Xor(ByVal A As HSet(Of T), ByVal _
B As HSet(Of T)) As HSet(Of T)
Dim result As New HSet(Of T)(A)
result.SymmetricExceptWith(B)
Return result
End Operator
' Subset.
Public Shared Operator <(ByVal A As HSet(Of T), ByVal B _
As HSet(Of T)) As Boolean
Return A.IsSubsetOf(B)
End Operator
' Superset.
Public Shared Operator >(ByVal A As HSet(Of T), ByVal B _
As HSet(Of T)) As Boolean
Return A.IsSupersetOf(B)
End Operator
' Contains element.
Public Shared Operator >(ByVal A As HSet(Of T), ByVal _
element As T) As Boolean
Return A.Contains(element)
End Operator
' Consists of a single element.
Public Shared Operator <(ByVal A As HSet(Of T), ByVal _
element As T) As Boolean
Return (A > element) AndAlso (A.Count = 1)
End Operator
End Class
|
|
The class starts with constructors that simply invoke the base class's constructors. These are needed so the main program can create an HSet and so the operators can create new HSets.
The binary (two operand) union, intersection, and XOR operators copy the first operand and then use the appropriate HashSet method to combine the copy with the second set.
The subset and superset operators simply invoke the HashSet class's IsSubsetOf and IsSupersetOf methods.
The "contains element" and "equals element" operators determine whether the set contains an element and whether it contains only the element. I would probably omit the second of these but the operators come in pairs so if you override the > operator then you must also override the < operator. (Alternatively you could have < throw an exception.)
|
|
|
|
|
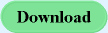 |
|
|
|