Title | Make a generic priority queue class in Visual Basic .NET |
Description | This example shows how to make a generic priority queue class in Visual Basic .NET. |
Keywords | classes, generic, generic classes, priority queue, PriorityQueue, Visual Basic .NET, VB.NET |
Categories | Algorithms, Software Engineering |
|
|
A generic class is similar to a regular class except it can take one or more parameters that are types. The class can then use the type to define variables and parameters with that type.
This example creates a generic PriorityQueue class that lets you associate a priority with objects of any given type. Its Enqueue method adds an item to the queue. Its Dequeue method returns the item with the largest priority from the queue and returns it.
The following code shows the generic PriorityQueue class.
|
|
Public Class PriorityQueue(Of T)
' The items and priorities.
Public Values As New List(Of T)()
Public Priorities As New List(Of Integer)()
' Return the number of items in the queue.
Public ReadOnly Property NumItems() As Integer
Get
Return Values.Count
End Get
End Property
' Add an item to the queue.
Public Sub Enqueue(ByVal new_value As T, ByVal _
new_priority As Integer)
Values.Add(new_value)
Priorities.Add(new_priority)
End Sub
' Remove the item with the largest priority from the
' queue.
Public Sub Dequeue(ByRef top_value As T, ByRef _
top_priority As Integer)
' Find the hightest priority.
Dim best_index As Integer = 0
top_priority = Priorities(0)
For i As Integer = 1 To Priorities.Count - 1
If (top_priority < Priorities(i)) Then
top_priority = Priorities(i)
best_index = i
End If
Next i
' Return the corresponding item.
top_value = Values(best_index)
' Remove the item from the lists.
Values.RemoveAt(best_index)
Priorities.RemoveAt(best_index)
End Sub
End Class
|
|
The (Of T) part of the class's declaration indicates that the class takes a type parameter named T. Inside the class, you can use the symbol T to represent the data type assigned to the class when the main program creates it.
The class starts by defining two lists to hold the items and their priorities. Notice how the code uses (Of T) to create a List containing whatever data type was used to create the PriorityQueue. The other list simply contains ints.
The NumItems property returns the number of items currently in the queue.
The Enqueue method adds an item to the Values list and its priority to the Priorities list. Notice how the new_item parameter has type T.
The Dequeue method finds the item with the largest priority, sets return values to return the item and its priority, and removes them from their lists.
The following code shows how the main program creates its PriorityQueue.
|
|
' The priority queue.
Private Queue As New PriorityQueue(Of String)()
|
|
After you create the queue, using it is easy. The following code shows how the program adds an item to it.
|
|
Queue.Enqueue(txtValue.Text, _
Integer.Parse(txtPriority.Text))
|
|
The following code shows how the program removes an item from the queue.
|
|
Dim top_value As String = ""
Dim top_priority As Integer
Queue.Dequeue(top_value, top_priority)
|
|
See the example code for other details.
The advantage of generic classes is that they can manipulate objects of any data type fairly easily. For example, this generic PriorityQueue class can hold strings, Employees, Orders, or just about anything else with which you might want to associate a priority.
The System.Collections.Generic namespace contains several useful generic classes including Dictionary, List, Queue (not a priority queue), Stack, and more.
|
|
|
|
|
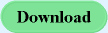 |
|