|
|
Title | Fill a shape drawn by the user with random lines in Visual Basic .NET |
Description | This example shows how to fill a shape drawn by the user with random lines in Visual Basic .NET. |
Keywords | graphics, lines, fill shape, user-drawn shape, random lines, random, example, example program, Windows Forms programming, Visual Basic .NET, VB.NET |
Categories | Algorithms, Graphics, Graphics, Graphics |
|
|
The user can click and draw to create the shape. Then the user releases the mouse, the program fills the shape with random line segments.
The following code shows how the program lets the user draw a shape.
|
|
' The points selected by the user.
Private ShapePoints As New List(Of Point)()
Private ShapePath As GraphicsPath = Nothing
Private LinesPath As GraphicsPath = Nothing
Private IsDrawing As Boolean = False
' Start drawing.
Private Sub Form1_MouseDown(ByVal sender As Object, ByVal e _
As System.Windows.Forms.MouseEventArgs) Handles _
Me.MouseDown
ShapePoints = New List(Of Point)()
ShapePoints.Add(e.Location)
IsDrawing = True
Refresh()
End Sub
' Continue drawing.
Private Sub Form1_MouseMove(ByVal sender As Object, ByVal e _
As System.Windows.Forms.MouseEventArgs) Handles _
Me.MouseMove
If Not IsDrawing Then Return
ShapePoints.Add(e.Location)
Refresh()
End Sub
' Finish drawing.
Private Sub Form1_MouseUp(ByVal sender As Object, ByVal e As _
System.Windows.Forms.MouseEventArgs) Handles Me.MouseUp
If Not IsDrawing Then Return
IsDrawing = False
' Generate the random lines to fill the shape.
GenerateLines()
Refresh()
End Sub
|
|
The MouseDown event handler starts the process. It saves the first point in the ShapePoints list and sets IsDrawing to true.
The MouseMove event handler adds a new point to ShapePoints if IsDrawing is true.
The MouseUp event handler calls GenerateLines if IsDrawing is true. The following code shows how GenerateLines works.
|
|
' Generate the random lines to fill the shape.
Private Sub GenerateLines()
If ShapePoints.Count < 3 Then
ShapePath = Nothing
LinesPath = Nothing
Return
End If
' Make the shape's path.
ShapePath = New GraphicsPath()
ShapePath.AddPolygon(ShapePoints.ToArray())
' Get the shape's bounds.
Dim bounds As RectangleF = ShapePath.GetBounds()
Dim xmin As Integer = CInt(bounds.Left)
Dim xmax As Integer = CInt(bounds.Right) + 1
Dim ymin As Integer = CInt(bounds.Top)
Dim ymax As Integer = CInt(bounds.Bottom) + 1
' Generate random lines.
LinesPath = New GraphicsPath()
Dim num_lines As Integer = CInt((bounds.Width + _
bounds.Height) / 16)
Dim rand As New Random()
Dim x1, y1, x2, y2 As Integer
For i As Integer = 1 To num_lines
x1 = rand.Next(xmin, xmax)
y1 = ymin
x2 = rand.Next(xmin, xmax)
y2 = ymax
LinesPath.AddLine(x1, y1, x2, y2)
x1 = xmin
y1 = rand.Next(ymin, ymax)
x2 = xmax
y2 = rand.Next(ymin, ymax)
LinesPath.AddLine(x1, y1, x2, y2)
Next i
End Sub
|
|
This code creates a new GraphicsPath object named ShapePath to represent the shape drawn by the user. It gets the bounds of the path. It then generates random line segments connecting the bounding rectangle's left and right edges, and connecting the bounding rectangle's top and bottom edges.
The following code shows how the program draws the filled shape.
|
|
' Draw the shape.
Private Sub Form1_Paint(ByVal sender As System.Object, ByVal _
e As System.Windows.Forms.PaintEventArgs) Handles _
MyBase.Paint
' Draw the shape.
If IsDrawing Then
' Draw the lines so far.
If ShapePoints.Count > 1 Then
e.Graphics.DrawLines(Pens.Green, _
ShapePoints.ToArray())
End If
Else
' Fill and outline the finished shape.
If ShapePath IsNot Nothing Then
e.Graphics.FillPath(Brushes.LightGreen, _
ShapePath)
e.Graphics.DrawPath(Pens.Green, ShapePath)
' Fill with the lines.
e.Graphics.Clip = New Region(ShapePath)
e.Graphics.DrawPath(Pens.Green, LinesPath)
End If
End If
End Sub
|
|
If the user is currently drawing, the program draws the shape so far as a series of lines.
If the user is not currently drawing, the program fills the shape's path and outlines it. Next it sets the Graphics object's clipping region to the path the user drew. It then draws the lines, which are clipped by the clipping region.
|
|
|
|
|
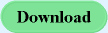 |
|
|
|