|
|
Title | Calculate how long it will take to pay off a credit card by making minimum payments in Visual Basic .NET |
Description | This example shows how to calculate how long it will take to pay off a credit card by making minimum payments in Visual Basic .NET. |
Keywords | mathematics, algorithms, finance, credit card, pay off credit card, minimum payments, example, example program, Windows Forms programming, Visual Basic .NET, VB.NET |
Categories | Algorithms |
|
|
For each month the program:
- Calculates the payment first. This is either a percent of the balance or the minimum amount, whichever is greater.
- Calculates interest after the payment is calculated but before it is subtracted from the balance.
- Adds the interest to the balance.
- If the payment is greater than the balance, the payment is set equal to the balance.
- Subtracts the payment from the balance.
- Displays the results for the month.
This method agrees well with the calculator at Bizrate.com.
The following code does all the work.
|
|
' Calculate the payments.
Private Sub btnGo_Click(ByVal sender As System.Object, ByVal _
e As System.EventArgs) Handles btnGo.Click
' Get the parameters.
Dim balance As Decimal = _
Decimal.Parse(txtInitialBalance.Text, _
NumberStyles.Any)
Dim interest_rate As Decimal = _
Decimal.Parse(txtInterestRate.Text.Replace("%", "")) _
/ 100
Dim payment_percent As Decimal = _
Decimal.Parse(txtPaymentPercent.Text.Replace("%", _
"")) / 100
Dim min_payment As Decimal = _
Decimal.Parse(txtMinPayment.Text, NumberStyles.Any)
interest_rate /= 12
txtTotalPayments.Clear()
Dim total_payments As Decimal = 0
' Display the initial balance.
lvwPayments.Items.Clear()
Dim new_item As ListViewItem = _
lvwPayments.Items.Add("0")
new_item.SubItems.Add("")
new_item.SubItems.Add("")
new_item.SubItems.Add(balance.ToString("c"))
' Loop until balance == 0.
Dim i As Integer = 1
Do While (balance > 0)
' Calculate the payment.
Dim payment As Decimal = balance * payment_percent
If (payment < min_payment) Then payment = _
min_payment
' Calculate interest.
Dim interest As Decimal = balance * interest_rate
balance += interest
' See if we can pay off the balance.
If (payment > balance) Then payment = balance
total_payments += payment
balance -= payment
' Display results.
new_item = lvwPayments.Items.Add(i.ToString())
new_item.SubItems.Add(payment.ToString("c"))
new_item.SubItems.Add(interest.ToString("c"))
new_item.SubItems.Add(balance.ToString("c"))
' Process the next month.
i += 1
Loop
' Display the total payments.
txtTotalPayments.Text = total_payments.ToString("c")
End Sub
|
|
To model a fixed payment (for example, $200 per month), set Payment % to 0 and set Min Payment to the monthly amount you want to pay.
Note that if you don't rack up this big debt and you put the payments in a savings account or some other investment, then compound interest will work in your favor instead of against you and you'll make money instead of paying out a lot of interest. For an example that calculates compound interest with a monthly investment, see Calculate the future value of a monthly investment with interest in Visual Basic .NET.
Suppose you want to buy something for $5,000.00. Using this example and the default settings, you end up paying $8,109.24 over 138 months. That's an average payment of $58.76, although in fact you pay a lot more in the beginning because your minimum payment is larger when the balance is bigger.
Instead of putting the $5,000.00 on your credit card, suppose you put $60.00 per month in an account that pays 7.00% interest. After only 69 months you would have saved $5,079.20. You would have enough for the $5,000.00 purchase with a little left over in half the time it would have taken you to pay off the credit card debt and with smaller payments initially!
Of course, there are a couple of catches. First, can you find an investment that pays 7.00% these days? If you only get a 1.00% return (which is believable even now), you still raise $5,025.61 in 81 months. That's not as fast but it's still a lot faster than paying off the credit card debt and with smaller contributions.
The moral is that you can save a lot of money if you save up for something instead of buying it on credit. You'll save the interest payments and be finished saving sooner than you would be finished paying off credit card debt.
|
|
|
|
|
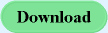 |
|
|
|